Representational State Transfer (REST) has become the standard architectural style for designing networked applications and services. RESTful APIs provide a flexible and scalable approach to building web services that can be consumed by various clients. In this article, we will explore the fundamentals of REST, its architectural principles, and best practices for designing and consuming RESTful APIs.
What is REST?
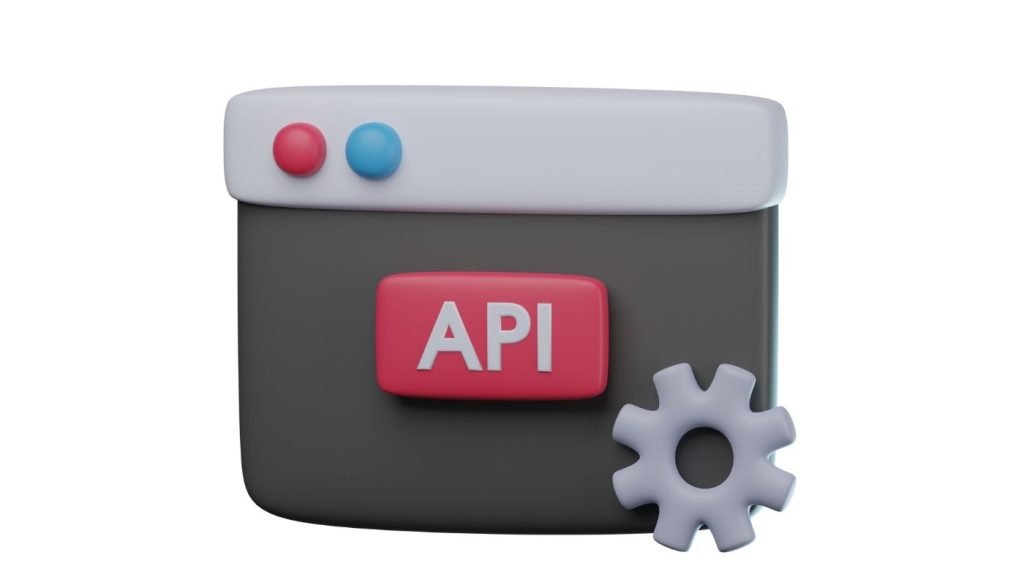
REST is a software architecture style that enables applications to be built in a distributed manner on top of the web. REST stands for Representational State Transfer and is an architectural style that is used to design web services. REST is not a standard, but a set of principles that define how a web service can be designed using the HTTP protocol. REST is based on the concept of resources that can be accessed using Uniform Resource Identifiers (URIs). A URI is a unique identifier for a resource, and can be used to access the resource using the HTTP protocol. The HTTP protocol is used to request and send data to and from resources.
REST applications use the HTTP verbs to interact with resources. The HTTP verbs are used to request a resource, update a resource, delete a resource, or create a resource. The most common HTTP verbs are GET, POST, PUT, and DELETE. REST, short for Representational State Transfer, is an architectural style for designing networked applications. It was first introduced by Roy Fielding in his doctoral dissertation in 2000. RESTful APIs are built using the principles of REST and enable communication between servers and clients over the internet.
REST Architecture Principles
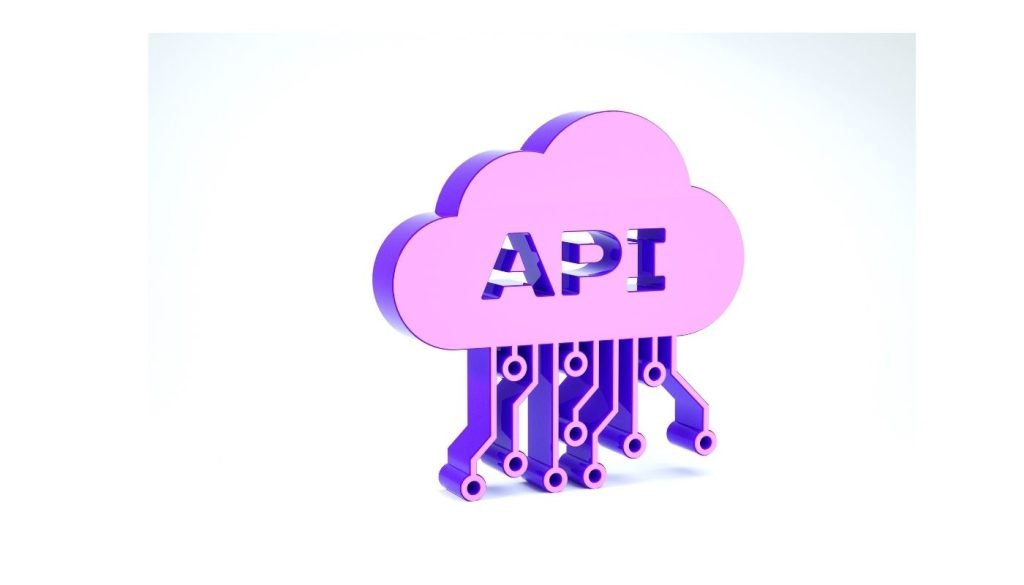
REST is built on a set of architectural principles that promote scalability, simplicity, and reliability. These principles include:
- – Stateless: Each request from a client to a server must contain all the necessary information to understand and process the request. The server should not store any client-related information between requests.
- – Client-Server Separation: The client and server are separate entities that communicate over a network. The client is responsible for the user interface, while the server handles data storage and processing.
- – Uniform Interface: RESTful APIs should have a standardized interface that allows clients to understand and interact with resources. This includes using HTTP verbs, proper resource design, and consistent response formats.
- – Cacheable: Responses from the server should be cacheable to improve performance and reduce the load on the server.
- – Layered System: RESTful APIs can be layered to improve scalability and flexibility. Each layer can provide additional functionality without affecting the overall system.
HTTP Verbs and CRUD Operations
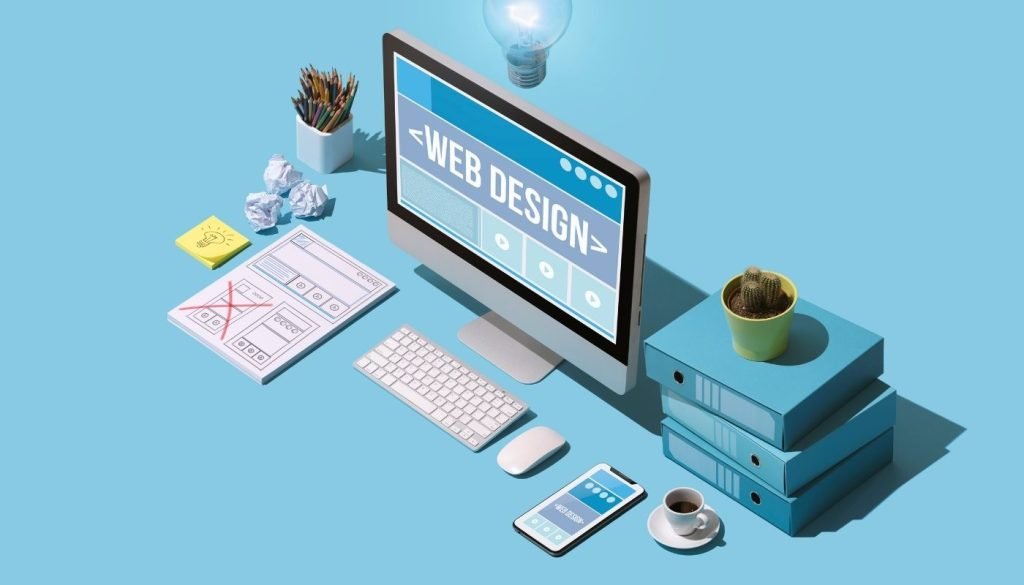
RESTful APIs use HTTP verbs to perform CRUD (Create, Read, Update, Delete) operations on resources. The commonly used HTTP verbs are:
– GET: Retrieves a resource or a collection of resources.
– POST: Creates a new resource.
– PUT: Updates an existing resource.
– DELETE: Deletes a resource.
Resource Design and URI Structure
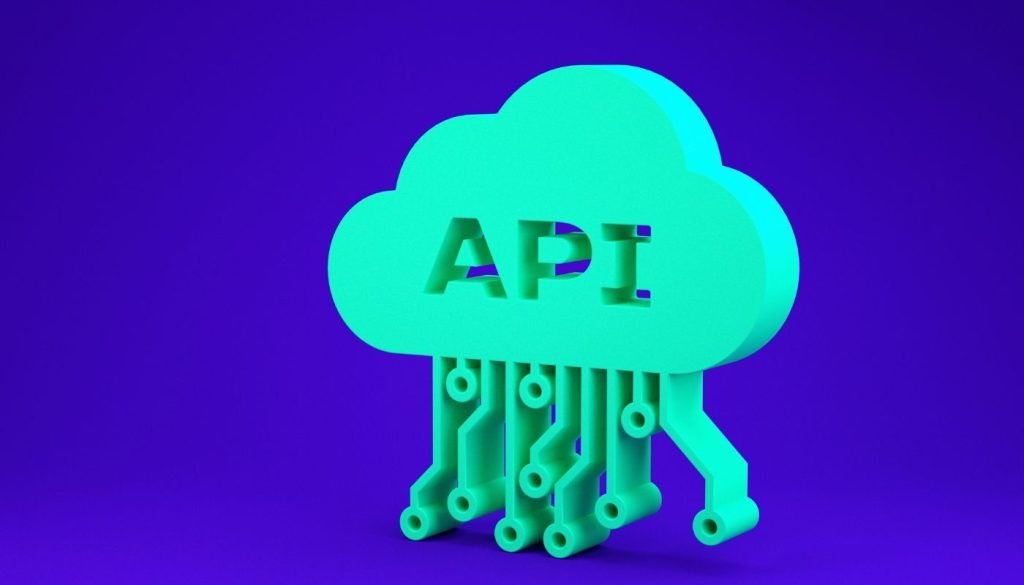
In RESTful APIs, resources are the key elements that clients interact with. Resources can represent entities such as users, products, or orders. Each resource should have a unique URI (Uniform Resource Identifier) that identifies it. The URI structure should be intuitive and follow best practices, including the use of nouns instead of verbs and avoiding complex hierarchies.
Request and Response Formats
RESTful APIs use various formats for request and response payloads, including JSON (JavaScript Object Notation) and XML (eXtensible Markup Language). JSON has become the preferred format due to its simplicity, lightweight nature, and easy integration with JavaScript-based clients. There are a variety of request and response formats used in web services. The most common formats are XML and JSON.
XML: XML (eXtensible Markup Language) is a standard data format used in web services. XML is a text-based format that uses tags to mark up data. XML is a widely used format, and most web services support XML.
JSON: JSON (JavaScript Object Notation) is a lightweight data format used in web services. JSON is a text-based format that uses JavaScript-like syntax to mark up data. JSON is a newer format, and is not as widely used as XML. However, it is becoming more popular because it is lightweight and easy to use.
Pagination and Filtering
API pagination and filtering allows developers to get the most out of their RESTful APIs. Pagination enables you to get a limited number of results at a time, while filtering allows you to specify the criteria for the results that are returned. In this article, we’ll take a look at how to use pagination and filtering with the popular Restful API framework, Express. API pagination is the process of returning a limited number of results at a time from an API. This is useful when you need to return a large number of results, as it prevents the API from returning all of the results at once, which can overload the server and cause performance issues.
To enable pagination in an Express API, you need to use the pagination middleware. The pagination middleware takes two parameters: the maximum number of results to return per page, and the number of pages to return. Here’s an example of how to use the pagination middleware, When dealing with large collections of resources, pagination and filtering mechanisms should be implemented to improve performance and reduce network traffic. Pagination allows clients to retrieve resources in smaller chunks, while filtering enables clients to specify criteria for resource retrieval.
Authentication and Authorization
To secure RESTful APIs, authentication and authorization mechanisms should be implemented. Authentication verifies the identity of the client, while authorization determines the client’s access rights to specific resources or operations. Common authentication mechanisms include API keys, tokens, and OAuth.
Error Handling and Status Codes
RESTful APIs should provide meaningful error messages and appropriate HTTP status codes to indicate the success or failure of a request. Standard HTTP status codes like 200 (OK), 404 (Not Found), and 500 (Internal Server Error) should be used consistently.
Versioning and Compatibility
As APIs evolve over time, versioning mechanisms should be implemented to ensure backward compatibility. Versioning can be done through URL path parameters, custom headers, or query parameters.
Caching and Performance Optimization
Caching is an essential technique for improving the performance and scalability of RESTful APIs. It reduces the load on the server by storing frequently accessed resources or responses in a cache. HTTP caching headers like Cache-Control and ETag can be used to control caching behavior.
HATEOAS and Hypermedia Controls
Hypermedia as the Engine of Application State (HATEOAS) is a principle that adds hyperlinks to API responses, enabling clients to navigate through the API dynamically. Hypermedia controls provide clients with the necessary information to discover and interact with related resources.
API Documentation and Testing
Well-documented APIs are crucial for developers to understand how to consume them effectively. API documentation should include information about resource endpoints, request and response formats, authentication requirements, error handling, and usage examples. Automated testing tools like Postman or Swagger can be used to validate API functionality.
API Security and Rate Limiting
API security should be a top priority when building RESTful APIs. Measures like encryption, input validation, and role-based access control help protect sensitive data. Rate limiting mechanisms prevent abuse and ensure fair usage of API resources by imposing limits on the number of requests a client can make within a certain time frame.
API Lifecycle Management
RESTful APIs have a lifecycle that spans from design and development to deployment and maintenance. Proper management of this lifecycle involves version control, change management, monitoring, and continuous improvement. PI lifecycle management is the process of managing the life of an API, from its inception to its retirement. This includes planning and designing the API, publishing it, managing it and eventually retiring it.
API lifecycle management is important because it ensures that APIs are well-managed and meet the needs of both developers and businesses. It also helps to ensure that APIs are properly documented and maintained, and that they are retired when they are no longer needed. There are several steps in the API lifecycle management process, including:
- Planning and designing the API
- Publishing the API
- Managing the API
- Retiring the API
- Planning and designing the API
The first step in API lifecycle management is planning and designing the API. This includes deciding what the API will do, what it will look like, and how it will be used. It’s important to plan and design the API properly so that it can be efficiently managed and used. API design is important because it determines how the API will function. The design should be clear and concise, and it should be easy to understand and use. The design should also be consistent with the overall goals of the API.
API management is important because it helps ensure that the API is used effectively and efficiently. It also helps to ensure that the API meets the needs of the users. API management can also help to improve the overall quality of the API.
Tools and Frameworks for Building RESTful APIs
Numerous tools and frameworks simplify the development and deployment of RESTful APIs. Popular options include Node.js with Express, Django Rest Framework, Ruby on Rails, and Spring Boot. There are a number of tools and frameworks that can be used for building RESTful APIs. In this blog post, we will discuss some of the most popular options.
First, let’s take a look at some of the most popular HTTP frameworks for building APIs. One of the most popular options is the Express framework for Node.js. Express provides a number of features for building APIs, including routing, middleware, and templating. Another popular option is the Spring Framework for Java. The Spring Framework provides a number of features for building RESTful APIs, including dependency injection, annotations, and a template engine.
Another important consideration when building APIs is the choice of data store. There are a number of options for data stores, including relational databases, NoSQL databases, and key-value stores. A few popular options for NoSQL databases include MongoDB and Cassandra. A few popular options for key-value stores include Redis and memcached.
Conclusion
RESTful APIs have revolutionized web services by providing a scalable and flexible approach to building and consuming networked applications. Understanding the principles and best practices discussed in this article will help you design robust and efficient APIs that meet the needs of your clients. Remember to consider factors like resource design, request and response formats, authentication, error handling, and performance optimization to ensure the success of your RESTful API projects